lazy.nvim is a modern plugin manager for Neovim.
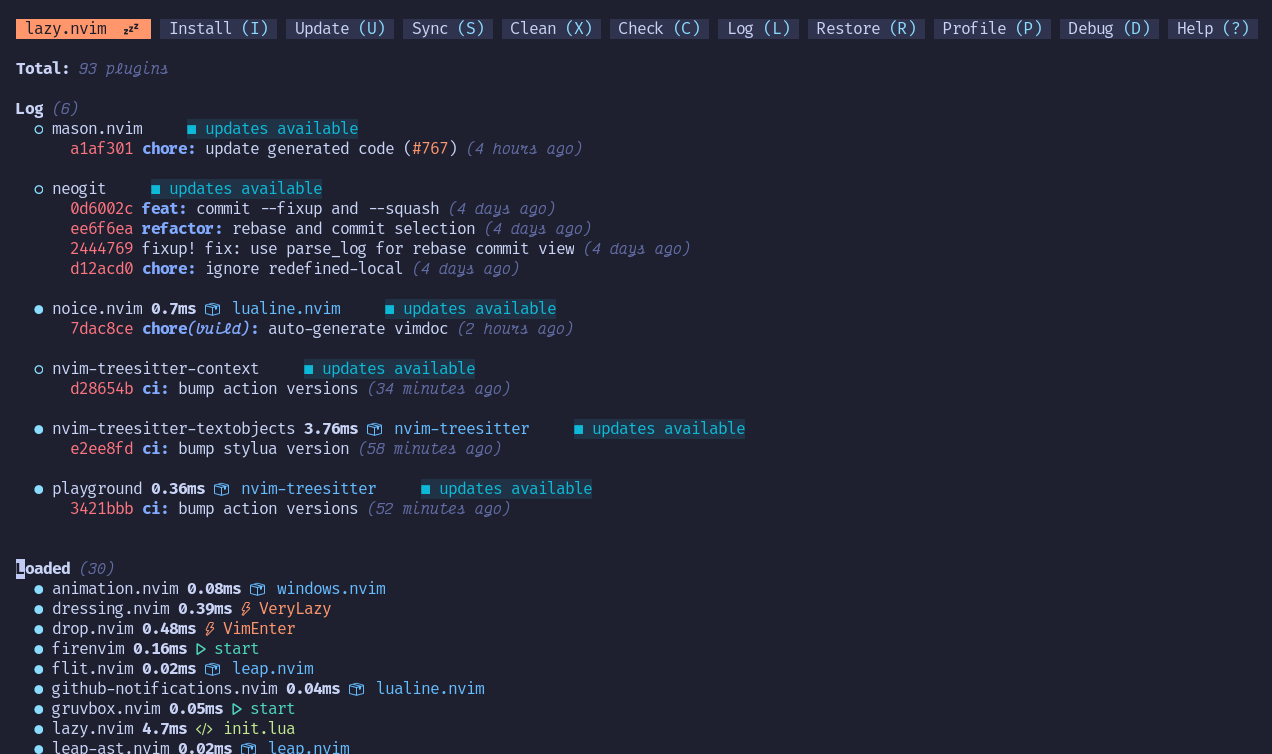
โจ Features
- ๐ฆ Manage all your Neovim plugins with a powerful UI
- ๐ Fast startup times thanks to automatic caching and bytecode compilation of Lua modules
- ๐พ Partial clones instead of shallow clones
- ๐ Automatic lazy-loading of Lua modules and lazy-loading on events, commands, filetypes, and key mappings
- โณ Automatically install missing plugins before starting up Neovim, allowing you to start using it right away
- ๐ช Async execution for improved performance
- ๐ ๏ธ No need to manually compile plugins
- ๐งช Correct sequencing of dependencies
- ๐ Configurable in multiple files
- ๐ Generates helptags of the headings in
README.md
files for plugins that don't have vimdocs
- ๐ป Dev options and patterns for using local plugins
- ๐ Profiling tools to optimize performance
- ๐ Lockfile
lazy-lock.json
to keep track of installed plugins
- ๐ Automatically check for updates
- ๐ Commit, branch, tag, version, and full Semver support
- ๐ Statusline component to see the number of pending updates
- ๐จ Automatically lazy-loads colorschemes
โก๏ธ Requirements
- Neovim >= 0.8.0 (needs to be built with LuaJIT)
- Git >= 2.19.0 (for partial clones support)
- a Nerd Font (optional)
- luarocks to install rockspecs.
You can remove
rockspec
from opts.pkg.sources
to disable this feature.
๐ Getting Started
Check the documentation website for more information.